Statistic
Display large centered text or variable information with a subtitle.
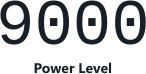
Usage
import { Statistic } from '@electricui/components-desktop-blueprint' <Statistic accessor ="boiler_w" label ="Heater Power" />
When using multiple statistics in the same area, wrap them in a <Statistics>
block.
import { Statistic , Statistics } from '@electricui/components-desktop-blueprint' <Statistics > <Statistic accessor ="boiler_power" label ="Heater Power" /> <Statistic accessor ="boiler_temp" label ="Fluid Temperature" /></Statistics >
The precision of floating point numbers is controllable. By default a Printer will render integers without decimal places 128, and floats with two decimal places 3.14.
<Statistic accessor="boiler_temp" label="Fluid Temperature" precision={4}/>
Prefix & Suffix Text
For variables with a unit or quantity intended alongside the value, the prefix
and suffix
properties provide a customisable string which appends to the rendered variable.

<Statistic accessor ="errorPPM" label ="ppm Error" prefix ="±"/> <Statistic accessor ="tempC" label ="Conductor Temperature" suffix ="°C"/>
Colours
The colour of the label can controlled with the color
property. This example imports a colour from a pre-made set, useful for maintaining consistency.

import { Colors } from '@blueprintjs/core' <Statistics > <Statistic accessor ="kg_potato" label ="kg ready" color ={Colors .BLUE3 } /> <Statistic accessor ="cook_timer" label ="minutes past due" color ={Colors .RED3 } /> <Statistic label ="Meals this hour" accessor ="meals_fin_h" color ={Colors .GREEN3 } /></Statistics >
Use #AABBCC
hex HTML colour codes, or rgb( 255, 60, 10)
rgb channels to set a custom colour. Use an online colour picker if you need help.

<Statistics > <Statistic accessor ="medal_cnt" label ="medals stamped" color ="rgb(183,149,60)" /> <Statistic accessor ="o2_missing" label ="seconds without oxygen" color ="#905eac" /></Statistics >
Colours can apply across child Statistic
components by setting the color
property on the <Statistics>
block wrapping them.
Colours applied to individual Statistic
components will override the group setting.

<Statistics color ="#0b3d91"> <Statistic accessor ="runtime" label ="hours elapsed" /> <Statistic accessor ="distance" label ="distance covered" color ="#fc3d21" /> <Statistic accessor ="fuel" label ="seconds of fuel" /></Statistics >
Layout
When positioning statistics alongside each other, centering and evenly spacing is often desired. If a Statistic
component is a direct child of a Statistics
wrapper, they will space evenly.
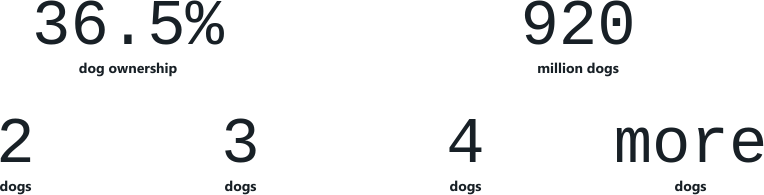
<Statistics > <Statistic value ="36.5%" label ="dog ownership" /> <Statistic value ="920" label ="million sensor" /></Statistics >
If the Statistic
components wrapped in another component, the spacing will not happen automatically and the inGroupOf
property must specify their spacing.
const WrappedStatistic = (props : WrapperProps ) => ( <Statistic accessor ={props .sensor } label ="dogs" inGroupOf ={4} />) const OverviewPage = () => ( <React .Fragment > <Statistics > <WrappedStatistic sensor ="laundry" /> <WrappedStatistic sensor ="bedroom" /> <WrappedStatistic sensor ="pool" /> <WrappedStatistic sensor ="lawn" /> </Statistics > </React .Fragment >)
Reversing the vertical ordering
To reverse the vertical ordering of the statistic, use the sub-components <Statistic.Value />
and <Statistic.Label />
. The <Statistic.Value />
takes either children or an accessor.
<Statistic > <Statistic .Label >Temp 1</Statistic .Label > <Statistic .Value suffix ="º">23</Statistic .Value ></Statistic >