Toast
Toasts provide in-window floating text notifications which are used indicate events.
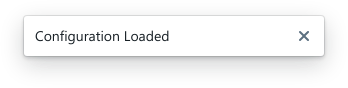
Usage
Toasts operate in two parts, the actual Toast
notification presented to the user, and the supporting code which creates Toast
by callback, typically triggered when specific messages are received from hardware.
As Toast usage is less-commonly required, it is not implemented in the template by default. See the implementation instructions in the next section.
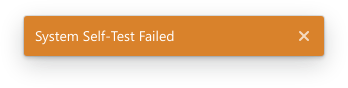
Once implemented, import the ToastConnector
component and ensure that it mounted in a persistent area. We recommend putting this alongside the template's persistent header, in the src/application/pages/DevicePages/index.tsx
file.
Implementation
Create a new file in src/application/Toaster.tsx
. This file will contain the code driving the display and dismissal logic, as well as the content displayed in the toasts.
This functionality is powered by the useMessageSubscription
hook. When an inbound packet with a message identifier error
is received, a callback is fired to create a toast using the packet's payload as the toast text.
import { useMessageSubscription } from '@electricui/components-core'import { Message } from '@electricui/core'import { Toaster, Position } from '@blueprintjs/core-core'import { React, useCallback } from 'react'
const ToastEmitter = Toaster.create({ className: 'error-toaster', position: Position.TOP,})export function ToastConnector() { const toastFunction = useCallback((message: Message) => { if (message.messageID === 'error') { ToastEmitter.show( { message: message.payload, intent: 'warning' }, message.payload, ) } }, []) useMessageSubscription(toastFunction) return null}
Adding additional toast types with varying styling can be done by calling Toaster.show
with different arguments for text, icons, colour, etc.
If the toast doesn't need to display dynamic text, the message
property can be supplied with a hardcoded string message: "Restarting System", ...
.
Template strings offer an elegant syntax to mix static and dynamic content.
ToastEmitter.show( { message: `The message was "${message.payload}"`, intent: 'warning' }, message.payload,)
TODO: See this recipe for a variation which catches identical inbound strings and displays repeated errors as a counter in the toast.
Auto-dismissal timeout
By default, a 5-second timeout will fade the toast after a period of time.
Pass a timeout in millseconds with the timeout
property.
If timeout: 0
is supplied in the Toaster.show()
call, the toast will persist until the user manually clears it.
Avoid 0-timeout Toasts!
We don't recommend this style of forced user interaction.
An
Overlay
with explicit interaction controls is a better solution for important events as the user's focus and cursor context are forced towards the relevant controls.
Toast Count & User Dismissal
Clamp the number of visible toasts by passing a number with the maxToasts
property.
Remove the users ability to dismiss the toast by setting the canEscapeKeyClear
property to canEscapeKeyClear: false
.
Toaster.show( { message: message.payload, maxToasts: 3, canEscapeKeyClear: false }, message.payload,)
Formatting
Intent Colouring
By default, Toast
will be styled unobtrusively to match the light or dark theme in use.
To draw attention to the Toast
, styling is available using the standard intent colouring system.
This can be specified as a string intent: 'warning'
or using the intent enum intent: Intent.DANGER
.
Icon
An Icon
can be displayed before the toast's text.
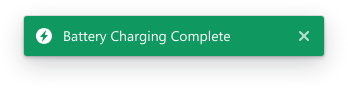
import { Icon } from '@blueprintjs/core-core'
Toaster.show( { message: message.payload, icon: 'offline', intent: 'success' }, message.payload,)
Position
The location inside the window can be controlled with the position
property. As with most Blueprint properties, this can be specified as a string position="top-left"
, or by using the Position.BOTTOM
enum.
Position Property | Description |
---|---|
top | Displays from the top of the window, center aligned |
top-left | Displays from the top-left corner of the window |
top-right | Displays from the top-right corner of the window |
bottom | Displays from the bottom edge of the window, center aligned |
bottom-left | Displays from the bottom-left corner of the window |
bottom-right | Displays from the bottom-right corner of the window |