Search Operators
closestSpacially
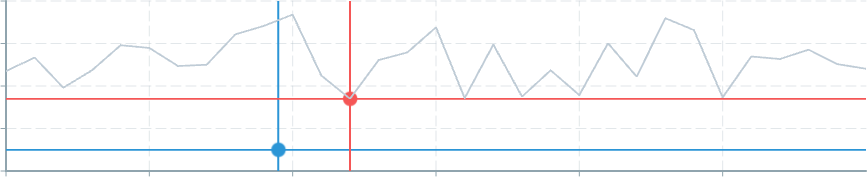
Finds the closest event in the first queryable to the search querable, sorted by geometric distance. In the screenshot above, the blue dot is at the search position, and the red dot shows the search result.
Input arguments are a pair of queryables, first is data, the second defines the search.
An unlimited, full density query is performed against the first queryable.
The most common use-case is driving annotations with mouse hover interactions.
import { useDataTransformer } from '@electricui/timeseries-react'import { closestSpatially } from '@electricui/dataflow'import { useMouseSignal } from '@electricui/components-desktop-charts' const [mouseSignal , captureRef ] = useMouseSignal () const closestEvent = useDataTransformer (() => { return closestSpatially (dataSource , mouseSignal , { queryablePositionAccessor : (data , time ) => ({ x : time , y : data }), })})
closestTemporally
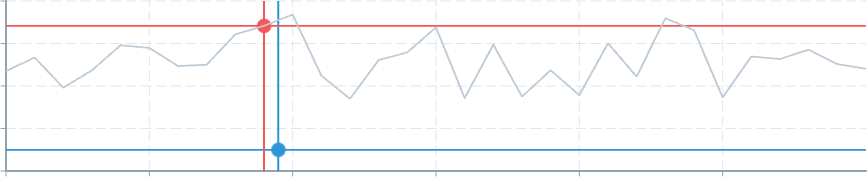
Finds the closest event in the first queryable, to the search querable, sorted by time. In the screenshot above, the blue dot is at the search position, and the red dot shows the search result.
Input arguments are a pair of queryables, first is data, the second defines the search.
In this code example, the accessor selects the time component (x
) of the mouseSignal
.
import { useDataTransformer } from '@electricui/timeseries-react'import { closestTemporally } from '@electricui/dataflow'import { useMouseSignal } from '@electricui/components-desktop-charts' const [mouseSignal , captureRef ] = useMouseSignal () const closestEvent = useDataTransformer (() => { return closestTemporally (dataSource , mouseSignal , data => data .x )})