ChartPauseButton
ChartPauseButton provides a quick and simple way to temporarily freeze the domain of a ChartContainer.
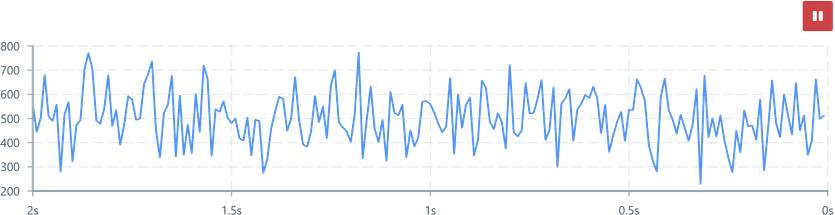
Usage
ChartPauseButton
is a pre-styled Blueprint button component. Internally, it toggles between the useChartPause()
callbacks pause()
and resume()
.
A sensible location is above the chart being paused.
import { ChartPauseButton } from '@electricui/components-desktop-blueprint-timeseries'
<div style ={{ display : 'flex', flexDirection : 'row', justifyContent : 'end' }}> <ChartPauseButton /></div > <ChartContainer > <LineChart dataSource ={adcDS } /> <TimeAxis /> <VerticalAxis /> <RealTimeDomain window ={10000} /></ChartContainer >
Domain ID
By specifying a domain identifier string, the pause behaviour can impact specific components:
ChartContainer
instances with a matching domain identifier,- Components wrapped by the
DomainWrapper
component with a matching identifier.
<ChartPauseButton domainID ='scope'/> <ChartContainer > <LineChart dataSource ={adcDS } /> <TimeAxis /> <VerticalAxis /> <RealTimeDomain window ={10000} domainID ='scope' /></ChartContainer >
Custom Styling
While additional Button
styling properties can override the default appearance, we recommend building your own button using the underlying useChartPause
API.
import React , { useCallback , useMemo } from 'react' import { Button , ButtonProps , Icon , Intent } from '@blueprintjs/core'import { useChartPause } from '@electricui/components-desktop-charts'import { IconNames } from '@blueprintjs/icons' interface StyledChartPauseButton extends ButtonProps { domainID ?: string} export function StyledChartPauseButton (props : StyledChartPauseButton ) { const { pause , resume , isPaused } = useChartPause (props .domainID ) if (isPaused ) { return <Button intent ={Intent .PRIMARY } large icon ={IconNames .PLAY } {...props } onClick ={() => resume ()} /> } else { return <Button intent ={Intent .WARNING } large icon ={IconNames .PAUSE } {...props } onClick ={() => pause ()} /> }}