DataSourcePrinter
Use DataSourcePrinter to render Dataflow stream values.
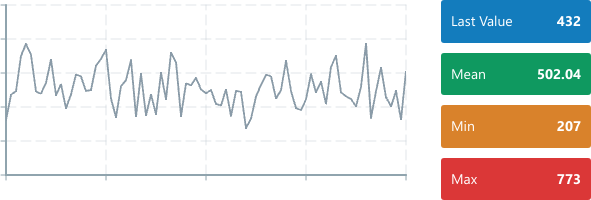
Usage
DataSourcePrinter
is different from the standard Printer
component - instead of subscribing to the state tree, it receives updates directly as the DataSource emits events.
Use it to display transformed data, query results, and alongside interactive charts as a legend.

import { DataSourcePrinter } from '@electricui/components-desktop-charts'import { useMessageDataSource } from '@electricui/core-timeseries' const OverviewPage = () => { const sensorDS = useMessageDataSource ('temp') return ( <> <DataSourcePrinter dataSource ={sensorDS }/> </> )}
Standard accessor
syntax allows for selection of specific member fields in the DataSource. A null
can be returned to set the DataSourcePrinter
to its defaultValue
.

<DataSourcePrinter dataSource ={sensorDS } accessor ={data => data .humidity }/> %RH
Stringification
The accessor
should only be used for picking particular pieces of data out of the event.
The formatter
prop can be used for formatting that data as a string. Using the JSON.stringify
method, object events can be printed as strings directly. This can be useful as a debug tool when working with complex Transformers.

<DataSourcePrinter dataSource ={sensorDS } formatter ={data => JSON .stringify (data )} />
Authoritative Domain Binding
By default, the DataSourcePrinter
will query data with the domain that has the longest window length.
This can be set to a specific domain with the domainID
prop on each DataSourcePrinter
component.
<ChartContainer > {/* */} <RealTimeDomain window ={5000} domainID ="primary" /></ChartContainer ><ChartContainer > {/* */} <RealTimeDomain window ={10000} domainID ="secondary" /></ChartContainer > <DataSourcePrinter dataSource ={sensorDS } domainID ="primary"/>
Alternatively, a DomainWrapper
can be used to set the authoritative domain for a group of components. The DomainWrapper
must include the chart providing the domain. In the example below, both DataSourcePrinter
s will use the primary
domain.
<DomainWrapper domainID ="primary"> <ChartContainer > {/* */} <RealTimeDomain window ={5000} domainID ="primary" /> </ChartContainer > <ChartContainer > {/* */} <RealTimeDomain window ={10000} domainID ="secondary" /> </ChartContainer > <DataSourcePrinter dataSource ={sensorDS } /> <DataSourcePrinter dataSource ={tempDS } /></DomainWrapper >
Reducing the update rate
The limitUpdateRate
prop accepts a number of milliseconds to wait between renders. It will always eventually render the latest data. By default, this is set to the update rate of the screen.
<DataSourcePrinter dataSource ={sensorDS } limitUpdateRate ={100} // update at 10Hz, at most./>
Formatting
Placeholder Text
The defaultValue
prop accepts a string to display when no value has been received.
The defaults to a blank string.

<b >Without defaultValue:</b ><DataSourcePrinter dataSource ={dataSource } /><b >With defaultValue:</b ><DataSourcePrinter dataSource ={dataSource } defaultValue ="Sensor Error" />
Precision
Like the normal Printer
component, the precision
property accepts a number to specify the number of decimal points.
The default precision for floats is two decimal places.
This only applies to values typed as
number
. It is ignored if theformatter
prop is set.

<DataSourcePrinter dataSource ={tempDS } precision ={0} /><DataSourcePrinter dataSource ={tempDS } precision ={1} /><DataSourcePrinter dataSource ={tempDS } precision ={2} /><DataSourcePrinter dataSource ={tempDS } precision ={4} /><DataSourcePrinter dataSource ={tempDS } precision ={8} />
Styling
Pass CSS to the inline span element with style
.
<DataSourcePrinter dataSource ={tempDS } style ={{ fontSize : '4em', fontWeight : "bold", color : Colors .RED2 }} />
The style can be set dynamically based on the event with styleAccessor
. The defaultStyle
is used when either no data has been received, or a null
has been passed by the accessor
.
<DataSourcePrinter dataSource ={closestDs } accessor ={(data , time , tags ) => data .closest } style ={{ fontSize : "4em", fontWeight : "bold", }} defaultStyle ={{ color : Colors .GRAY3 }} styleAccessor ={(data , time , tags , accessed : number) => { if (accessed > 90) { return { color : Colors .RED3 } } else if (accessed > 50) { return { color : Colors .ORANGE3 } } else { return { color : Colors .BLUE3 } } }}/>