Maintenance Release
Released on 2025-04-28
New features
New visual look
The upgrade to Blueprint 5 brings an updated visual look to dark mode, with better WCAG 2.0 standard compliance, with additional minor improvements to light mode.
Chart Scissoring
Charts now use a single screen-sized WebGL canvas and dynamically track charts within the viewport. This removes the framebuffer restriction (of 16 charts) and reduces the 'boot up' time of charts. The following example has 24 charts, each displaying 1000 points, updating at 500Hz each.
Headless template DataFlow
The headless template now has APIs to receive connection metadata and device messages as a native DataSource. The timeSlice
API lets you perform incremental DataFlow computations while issuing imperative instructions to the hardware. The following example does a naive bandwidth test, while printing in-progress results to the terminal.
await report.timeSlice( async () => { await write('led_blink', 1) // start blinking await sleep(3_000) // wait 3 seconds await write('led_blink', 0) // stop blinking }, // calculate a series of statistics coalesce({ bpsIn: mean(bpsIn), // the mean incoming bandwidth vps: mean(vps), // the mean valid incoming packets ips: mean(ips), // the mean invalid incoming packets }), // Print the result live as data comes in. (res, time) => { return `With lit_time: ${opt}, received ${formatBandwidth(res.bpsIn)}, ${ res.vps ? Math.round(res.vps) : 'no' } valid PPS, ${res.ips ? Math.round(res.ips) : 'no'} invalid PPS` },)
➤ ┌ Finding eUI device...➤ └ Completed in 504ms➤ Found device 50018➤ Connected to device successfully➤ led_blink was set to 1. Checking if auto-send is setup.➤ Auto send validated, received 19970 messages.➤ With lit_time: 1000, received 18.30 Kib/s, 1042 valid PPS, no invalid PPS➤ With lit_time: 100, received 174.97 Kib/s, 9955 valid PPS, no invalid PPS➤ With lit_time: 50, received 349.91 Kib/s, 19598 valid PPS, no invalid PPS➤ With lit_time: 25, received 700.29 Kib/s, 39840 valid PPS, no invalid PPS➤ With lit_time: 10, received 1.71 Mib/s, 99595 valid PPS, no invalid PPS⠏ With lit_time: 1, received 5.70 Mib/s, 123183 valid PPS, no invalid PPS
Performance Improvements
The Charts and DataFlow systems have received a series of performance improvements:
- A zero-copy QueryableMessageIDProvider batch API for large message packets with bulk data.
- SIMD accelerated bounds checking.
- An Arena Allocator for all chart backends, vastly reducing memory usage of small annotations.
- Improved GPU data streaming, vastly reducing bandwidth requirements.
- Charts that don't use continuity or DataFlow bypass the system entirely, increasing performance.
- DataFlow advancement is now zero-copy. Interning buffers into DataFlow is also zero-copy in the majority of cases.
- Multiple requests to the same Queryable within a DataFlow plan are now cached.
Time-to-first-point has been reduced from ~100ms to ~75ms for the first chart, and ~25ms for each chart after that.
Our "million point benchmark" with a batch size of 1000 previously took 2 seconds to complete, averaging 492 thousand points per second, it now completes in ~160ms, averaging 6.2 million points per second.
We've created a new throughput benchmark to better show the amortization of startup costs, which renders 5x 20kHz charts at 100fps for an overall throughput of 10 million points per second.
Our DataFlow benchmarks have had an average of a 1.23x improvment at a batch size of 1, and a 1.06x improvement at a batch size of 1000.
Benchmark | Prev (1) | New (1) | Prev (1k) | New (1k) | Impr (1) | Impr (1k) |
---|---|---|---|---|---|---|
Raw Passthrough | 2,735k | 2,777k | 15,888k | 21,181k | 1.02x | 1.33x |
DataFlow Passthrough | 1,045k | 1,435k | 7,750k | 9,589k | 1.37x | 1.24x |
Mean | 837k | 1,076k | 5,348k | 5,602k | 1.29x | 1.05x |
Sum | 853k | 1,090k | 5,148k | 5,383k | 1.28x | 1.05x |
Count | 852k | 1,077k | 5,230k | 5,859k | 1.26x | 1.12x |
Standard Deviation | 849k | 1,059k | 5,520k | 5,454k | 1.25x | 0.99x |
Variance | 843k | 1,060k | 5,401k | 5,593k | 1.26x | 1.04x |
Root Mean Square | 846k | 1,062k | 4,060k | 5,494k | 1.25x | 1.35x |
Histogram | 864k | 1,059k | 6,187k | 6,374k | 1.23x | 1.03x |
Leaky Integrator | 985k | 1,276k | 7,223k | 7,374k | 1.30x | 1.02x |
Instantaneous Derivative | 992k | 1,271k | 7,056k | 7,299k | 1.28x | 1.03x |
Max | 335k | 422k | 894k | 1,017k | 1.26x | 1.14x |
Quantile | 364k | 410k | 744k | 769k | 1.13x | 1.03x |
Coalesce 3 Quantiles | 128k | 155k | 245k | 262k | 1.21x | 1.07x |
Min Max Mean Sum Count | 127k | 182k | 337k | 346k | 1.43x | 1.02x |
General
There are numerous general performance improvements.
- The Electric UI Binary Protocol decoder is now zero-copy.
- Number codecs now have zero-copy fast paths for arrays as long as alignment matches up.
- The COBS decoder is ~10x faster, utilising a look-up-table. It is now fuzz tested.
- The serialport internal pool size can now be tuned for increased performance.
Event rate statistics in the transport window are now more stable at high rates. For example here is the hello-blink example running at 100kHz.
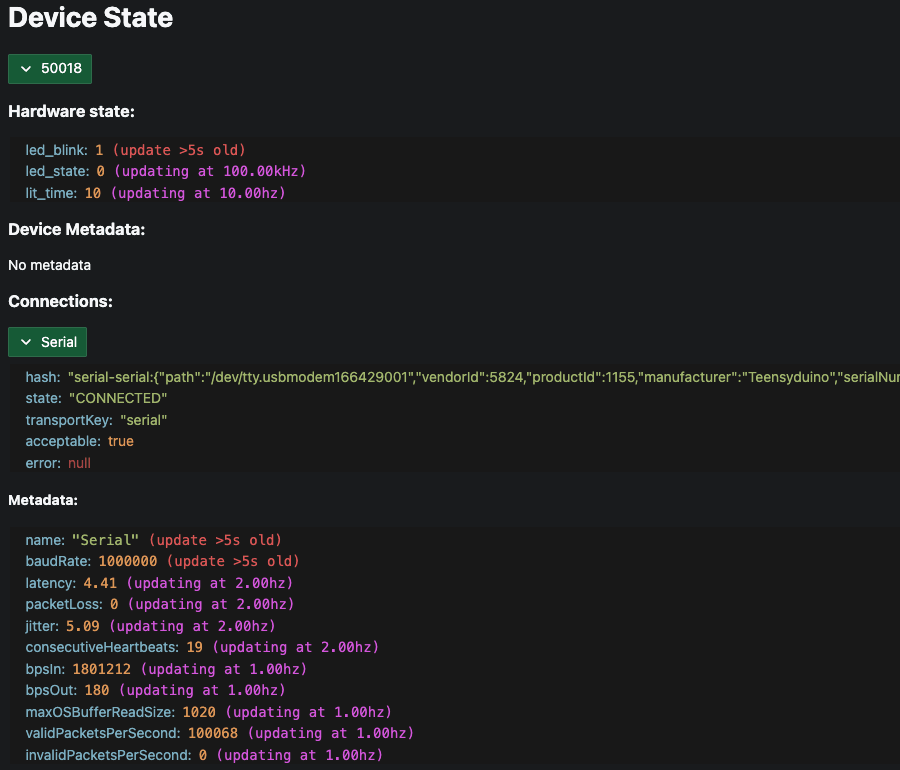
Several new connection statistics are now available, including:
- The maximum OS buffer size provided on read.
- How many valid / invalid packets per second are being decoded by the binary protocol decoder.
Breaking Changes
DeviceManager.stopPolling
has been removed in favour of cancelling theCancellationToken
passed topoll
.- In Blueprint 5, the COBALT colour was removed and replaced with CERULEAN
Versioning updates
- React 18
- ThreeJS v174
- Blueprint 5
- TypeScript 5.8.2
- Electron 35
Bugfixes
- Fixed acceptability of connection not reaching client on first boot
- Fixed EventCSVLogger using wrong time origin
- Fixed annotations pulling tiny window limits from maxItems
- Fixed out of order remote queryable frontier updates
- Fixed charts rendering blank axes
- Fixed coalesce operator not always receiving latest values on batch end
- Fixed fast updating, low batch size charts running out of buffer and 'squashing'
- Fixed sub-pixel sizing in charts causing bounds check errors
- Fixed garbage collection mangling the point buffer
- Fixed misaligned icons, popover library version
- Fixed closestTemporally emitting some searches out of order
- Gracefully handle identical timestamped hardware messages in the same stream
Update instructions
An automatic upgrade is available by running the following command in your template directory:
arc upgrade --template [email protected]
Feel free to contact support if you need any help upgrading.