IPC, PnP, Hooks
Released on 2020-10-18
Mojo Pipes for IPC communication
The internal IPC system has been rewritten to use the internal Mojo pipes system in Chromium instead of named pipes which had connection issues on Windows.
Yarn PnP
Package management is now handled by Yarn PnP, this reduces the dependency size on disk by several hundred megabytes. Template instantiation should also be faster.
The native dependency management has also been improved.
Incremental compilation for fast hot reloads
Various packages (including their CSS) have been moved into a 'vendor bundle' which will be compiled on first run of the program.
Hot reloads as a result will only have to compile against developer code, resulting in an order of magnitude faster builds.
Electron 8
Electron has been updated to version 8. V8 is now also v8. Node is now v12.13.0.
Window display delay
The UI windows will no longer be displayed before the transport process is ready to accept connections, improving perceived launch performance.
Improved message timestamping
Previously timing of Message arrival was timed by the UI thread. When the UI had 'jank', this would result in late times for incoming messages.
Messages are now timed by the transport thread, and that time is stored in the timestamp field of the metadata object.
Performance marks for time based debugging
Debugging connection behaviour can be difficult given its time dependent nature. Performance marks have now been added for several of the connection functions in order to better establish timing information between them.
Heartbeat exponential backoff startup
The heartbeat metadata reporter will now use an exponential backoff startup sequence to establish initial heartbeat data upon connection. It can be configured with the exponentialBackoffStartup option.
Attachment delays for Arduinos
Some Arduinos will restart upon connection. If the developer doesn't wish to make hardware changes to prevent this, a new attachmentDelay option is available for the serial transport to delay connection for a period of time.
Setting this to 2000ms allows for most Arduinos to complete their restart sequence before Electric UI conducts search packets.
Strict typing of Developer State
Developer state can now be globally strictly typed in the typedState.ts file. Documentation here.
All functional accessors will now return correct types for fetched variables.
Many components also now use discriminated unions to ensure compile-time prop compliance where writers are required with functional accessors.
New writer API
The object based writer API has been removed and replaced with a functional writer API.
<Button writer={{ rgb: { red: 255 } }}>Set Red</Button>
...is now...
<Button writer={state => {state.rgb.red = 255}}>Set Red</Button>
This fixes several problems with deep merging, making the mutation semantics more obvious.
Arrays of structures are now supported with all components.
<Button writer={state => {state.rgb[32].red = 255}}>Set LED 32 to Red</Button>
This is a breaking change.
New hooks API
All internal components have been refactored to use a new hooks API. Performance has been greatly improved for UIs that have many components that don't update together often.
The 'other half' of the public hooks API has also been completed. Writing from a custom component to a device is now simplified. The main new hooks are:
useWriteStateuseCommitStateusePushMessageIDs
New accessor and writer documentation is available here, and all new hook documentation is available here.
Chart Annotations
Charts now support horizontal and vertical line annotations.
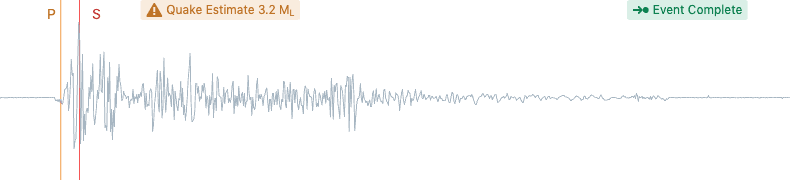
Docs are available here.
RollingStorage Manager
All consumers of the new DataSource API can have their events persist with the new RollingStorageRequest API. Docs are available here.
High performance Charts API
A from-scratch charting implementation has been developed. Documentation is available here.
Features include massively increased performance, achieving 165fps in any reasonable circumstances, the ability to plot a million points, the ability to consume ~100k Events per second.
Errata
2020-03-25
A bug was fixed with IPC message serialisation that resulted in some operations relating to cross process promises failing.
The pinning of the downstream dependency html-loader caused a bug that prevented installation, this is now resolved.
In some cases the heartbeat between the IPC would arrive in the UI window before the UI window received the location of the transport window, causing a race condition. This has now been resolved.
2020-03-28 Transport Manager Debug Interface Rework
The transport manager's debug interface has been reworked. It now imports from components-desktop-blueprint
instead of components-desktop
and as a result requires the Blueprint styling.
If you aren't using the Blueprint styling let us know.
2020-04-05 Heartbeats are no longer retried
Heartbeat messages are no longer automatically retried by the FIFO message queue. They are now considered 'one shot' messages.
Other 'one shot' messages can be added to the FIFO message queue with the oneShotMessageIDs
option.
This change will make the consecutive heartbeats counter more sensitive to delivery failures.
2020-04-15 Handshake Refactor
The handshake will now request items individually if it steps into the 'individual request mode'.
Handshake tests now cover 100% of lines, statements and branches within the module.
An Undefined MessageID Guard has been added to the template's default set of pipelines.
MessageIDs that have not been seen before, without type information from the hardware will explicitly error when sent to hardware.
This should guard against spelling mistakes in messageIDs.
The heartbeat startup sequence now immediately returns on a successful heartbeat, instead of waiting 2 seconds for heartbeat #2 to return (in the case of the default startup sequence).
2020-04-19 Slider twitch bug
Slider
will now hold optimistic state until the packet has either been explicitly confirmed, or in the case of no-ack packets, written, or a rejection or timeout.
This prevents sliders from displaying the 'old' hardware state for a frame after releasing them.
The Blueprint Electric UI components will now pin their write errors against the components in the React tree, allowing for greater ease of debugging.
2020-05-03 Device primary connection hooks
The new useProduceHint
hook can be used to send Hints from the user interface to the transport manager. For example it can be used to supply a websockets URI for the WS transport without any external hint producer.
The useDevicePrimaryConnectionHash
and the useDevicePrimaryConnectionTransportKey
hook have been added.
They can be used to find the connection hash and the transport key of the primary connection method of a device, respectively.
They use the CONNECTION_METADATA_RANK_KEY="connectionRank"
metadata key of the connection in order to determine the primary connection, with rank #0 being the primary connection.
2020-05-05 Connection improvements & Electron Builder dep removal
If a device has multiple connections available upon first connection, they will be raced instead of waiting for all to complete connection before starting the handshake procedure.
Wait For Reply calls can now optionally include a description that's calculated on request instead of the textual form of the closure used to validate incoming messages. This aids in debugging when the calls fail.
All internal usages of the Wait For Reply calls have been annotated with a debug message to help with tracking down message failures.
The timeseries dependency Pond.js relies on moment-timezone
. In our gradual effort to refactor the timeseries system timezone support has been removed.
This is a breaking change but should not affect anyone during regular usage of the timeseries API.
The Electron Builder dependency has been removed due to an upstream problem preventing installations on certain Windows platforms.
2020-07-18 Template Improvements
Serial comPaths in Linux that start with /dev/ttyS
will be filtered by default in the latest template.
The latest version of VSCode allows for a prompt to switch to the workspace version of TypeScript. This has been added to the template.
Electron v9.0.0 is now supported.
Monospace fonts are now the default for statistic values.
2020-09-20 Cancellation Tokens
Electron v9.1.0 is now supported.
The transport console will now open correctly.
Instead of timeouts, CancellationTokens are now used across the entire ElectricUI API surface.
They allow for hard deadlines for tasks, as well as user based cancellation at any point in the lifecycle.
const cancellationToken = new CancellationToken()cancellationToken.deadline(10_000)setTimeout(() => cancellationToken.cancel(), 5_000)
2020-09-29 Line Chart Thickness & Minor fixes
The device manager proxy has had some of it's methods refactored to match the signature of the real device manager.
Electron v10.1.3 is now supported.
LineChart
now supports lines thicker than 1px.
2020-09-30 New Codecs API
The codecs API has been refactored.
The old API received the entire message and a callback to push any amount of messages up the pipeline.
export type LEDSettings = { glowTime: number enable: number}export class LEDCodec extends Codec { filter(message: Message): boolean encode( message: Message<LEDSettings>, push: PushCallback<Message<Buffer>>) { }: void decode( message: Message<Buffer>, push: PushCallback<Message<LEDSettings | null>>, ): void}
The new API reduces the functionality to only transform a payload to and from a Buffer.
The Pipelines API still has the capability to have the forking behaviour that was available here if need be, but this vastly simplifies the usual use case.
export type LEDSettings = { glowTime: number enable: number}export class LEDCodec extends Codec { filter(message: Message): boolean encode(payload: LEDSettings): Buffer encode(payload: Buffer): LEDSettings}
2020-10-23 October Maintenance Bump
Yarn has been updated to v2.2.2.
React 17 is now supported.
Some minor internal package bumps, and bumps to versions in the template.
THREE will no longer complain that Scene has no dispose method.
Fixes a bug with polling when no devices are attached.
Multiple charts rendered by the same datasource will have their updates batched in the same React pass.
2020-11-14 November features update
The CodecPipeline can now optionally error if no codecs match.
ColorPicker
s can no longer be manipulated 'though' a modal over the top of them. Previously they didn't do a depth test and unintended behaviour resulted.
CSVLogger
components and hooks no longer use Electron's remote module for file dialog opening. They now have a dedicated IPC channel.
The Dropdown
component is now available.
The getDeviceAcceptableTransportKeys
hook can now be used to grab the acceptable transport keys for a device.
RadioButton
and NumberInput
have been refactored to use our hooks API internally.
TextInput
components now take a maxLength
property to limit the length of text enterable into the field.
yarn.lock
files will now be stable between operating systems. Prebuilt native dependencies will be fetched in a post-install step and their cache keys are now stored separately to the yarn.lock.
2020-11-19 November (late) improvements
The new resolver was grabbing webpack 5 instead of the required v4.
The shader used by LineChart
to increase the width of plotted lines has been refactored.
The RealTimeDomain
property window accepts an array of window timescales to allow the LineChart to automatically increase it's x-axis domain over longer periods.
It was incorrectly only using the smallest window in the array. It should now auto-range across the array values correctly.
Test coverage for dynamic RealTimeDomain window resizing to prevent regressions.
TimeAxis
(x axis) now supports a tickCount
property to explictly control the number of horizontally drawn ticks.
node-abi
updated for Electron 11.
The template had missing serialport types, which meant the transport-manager/config/serial.tsx
would throw linter warnings around baudRate configuration.
Allows custom control over the text displayed when no devices could be found during search. Pass a noDevicesText
string into the Connections
component to modify the default.
Removes automatic colour space management in Threejs
which caused an incorrect shift on input colours (as they were in the correct colourspace to begin with).
LineChart
lines now support opacity. The opacity
prop accepts a number from 0 to 1 to control line translucency.
Fixed a regression in the automatic colors functionality for LineChart
.
On the upcoming Electron v11 branch, NodeUSB wouldn't build, this has been rectified.
If a release on latest
is ahead of one on next
, the next
channel will use the latest
release.
Builds had a regression where the app-builder
binary wasn't marked as executable. This has been fixed.